반응형
C 언어로 Stack을 구현하는 코드
//#pragma warning(disable:4996)
#define STACK_SIZE 5
#include <stdio.h>
typedef int element;
element stack[STACK_SIZE];
int top = -1;
void PrintStack() { //스택값 출력
int i;
printf("\nSTACK 상태: \n");
for(i=STACK_SIZE-1; i>=0; i--){
printf("[%d]=%5d\n" , i, stack[i]);
}
}
void createStack(int n){ //스택 초기값 설정
int i;
for(i=0; i<n; i++){
stack[i]=-1;
}
printf("스택을 초기화하였습니다.\n");
PrintStack();
}
element pop(){ //스택 pop 연산
int popvalue;
if(top == -1) {
printf("Stack is Empty!! \n");
return 0;
}
else {
printf("stack[%d] output %d pop success! \n", top, stack[top]);
popvalue = stack[top];
stack[top--]=-1;
return popvalue;
}
}
void push(){ //스택 push 연산
element item;
printf("스택에 저장할 값을 입력하세요 : ");
scanf("%d",&item);
if(top >= STACK_SIZE-1){
printf("Stack is Full!! \n");
//return;
}
else {
stack[++top] = item;
printf("stack[%d] input %d : push success! \n", top, stack[top]);
}
}
void main() {
createStack(STACK_SIZE);
push();
PrintStack();
push();
PrintStack();
push();
PrintStack();
push();
PrintStack();
push();
PrintStack();
push();
pop();
PrintStack();
pop();
PrintStack();
pop();
PrintStack();
pop();
PrintStack();
pop();
PrintStack();
pop();
PrintStack();
printf("The End. \n");
}
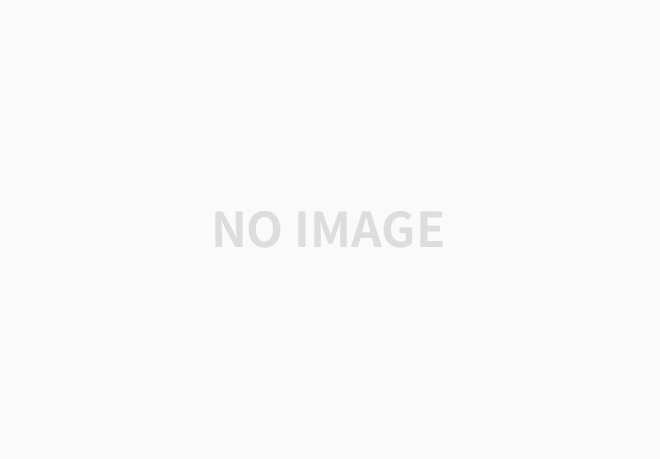
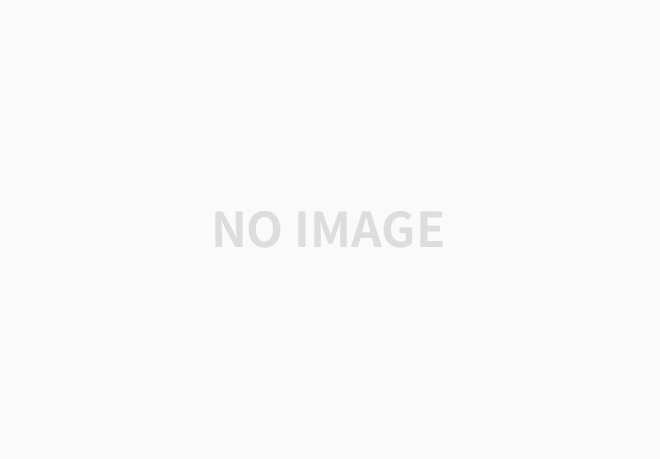
728x90
반응형
'Programming > Data Structure' 카테고리의 다른 글
[C++] 원형 연결 리스트 코드 구현 방법 Circular linked list (1) | 2020.09.11 |
---|---|
[C++] 이중연결리스트 코드 구현 방법 Doubly linked list (0) | 2020.09.10 |
[C++] 연결리스트 Linked list 코드 구현 방법 (0) | 2020.09.10 |
[C++] 큐 Queue 구현 방법 코드 (0) | 2020.09.09 |
[C++] 정수형 스택 STACK 구현 코드 (with 후위계산법) (0) | 2020.09.09 |